Bitcoin Clock ⌛
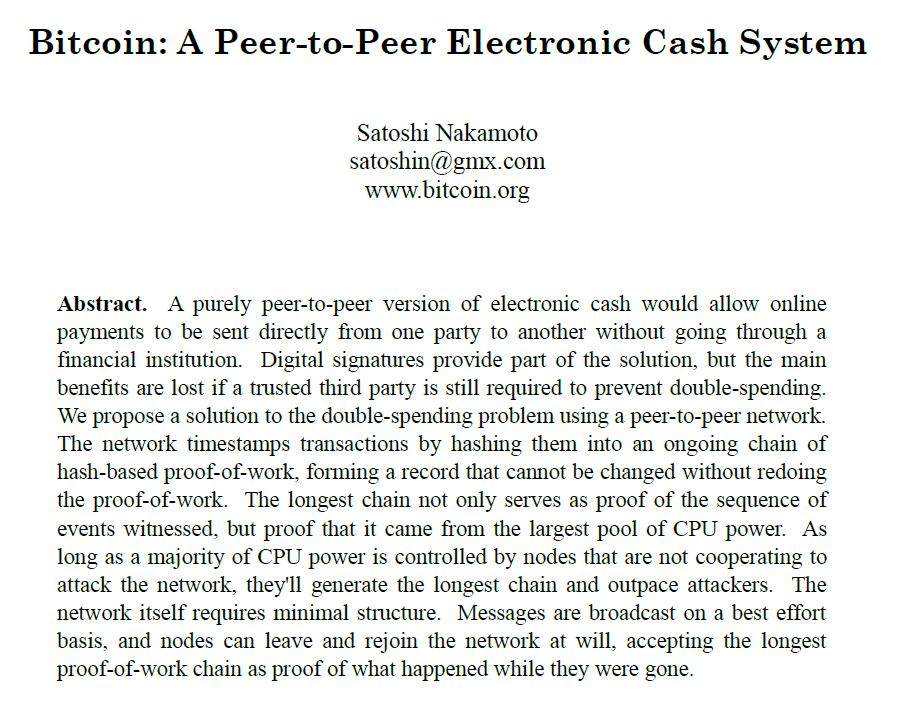
Prints the current Bitcoin block height to a tkinter window. Queries the blockchain every 45 seconds.
Block height clock
import time
import requests
from tkinter import *
from tkinter.ttk import *
def get_current_block_height():
url = "https://blockchain.info/latestblock"
response = requests.get(url)
data = response.json()
return data['height']
# Creating tkinter window
root = Tk()
root.title('Clock')
root.configure(bg='orange')
# Function to update the clock display
def clock():
current_block_height = get_current_block_height()
since_halving = current_block_height - 630000
next_halving = 840000 - current_block_height
block_height_string = str(current_block_height)
lbl.config(text= block_height_string)
# Update the labels for "Blocks since last halving" and "Blocks until next halving"
since_halving_string = "Blocks since last halving: "
until_halving_string = "Blocks until next halving: "
lbl_since_halving.config(text=since_halving_string + str(since_halving))
lbl_until_halving.config(text=until_halving_string + str(next_halving))
lbl.after(45000, clock)
# Styling the label widget for the block height
lbl = Label(root, font=('digital-7', 40, 'bold'),
background='orange',
foreground='white')
# Placing the block height label at the center of the tkinter window
lbl.pack(anchor='center')
# Styling the labels for "Blocks since last halving" and "Blocks until next halving"
lbl_since_halving = Label(root, font=('calibri', 12),
background='orange',
foreground='white',
anchor='w')
lbl_until_halving = Label(root, font=('calibri', 12),
background='orange',
foreground='white',
anchor='e')
# Placing the labels for "Blocks since last halving" and "Blocks until next halving"
lbl_since_halving.pack(side='left', padx=10, pady=5)
lbl_until_halving.pack(side='right', padx=10, pady=5)
clock()
root.mainloop()